# 7. Vue-resource 实现请求
官方文档
https://github.com/pagekit/vue-resource
CDN 引入
<script src="https://cdn.jsdelivr.net/npm/vue-resource@1.5.1"></script>
1
# 普通请求
下面是一个模拟请求示例
<!DOCTYPE html>
<html>
<head>
<title>vue-Resource</title>
<script src="./lib/vue/vue.js"></script>
<script src="./lib/vue/vue-resource.js"></script>
</head>
<body>
<div id="app">
<input type="button" value="GET请求" @click="requestApi('GET')" />
<input type="button" value="POST请求" @click="requestApi('POST')" />
</div>
</body>
<script>
// Vue 实例
var vue = new Vue({
el: '#app',
data: {},
methods: {
requestApi(typeName) { // 发起get请求
switch (typeName) {
case 'GET':
this.$http.get('http://localhost/test/login1').then(res => {
console.info(res)
}, res => {
console.info(res)
})
break;
case 'POST':
this.$http.post('http://localhost/test/login2', { value: {} }, {}).then(res => {
console.info(res)
}, res => {
console.info(res)
})
break;
}
}
}
})
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
# 英雄系统案例
# 前言
搭建了一个java的服务端,使用vue-resource进行请求,并封装vue-resource,实现英雄系统增加、删除、查询功能。
# 演示效果
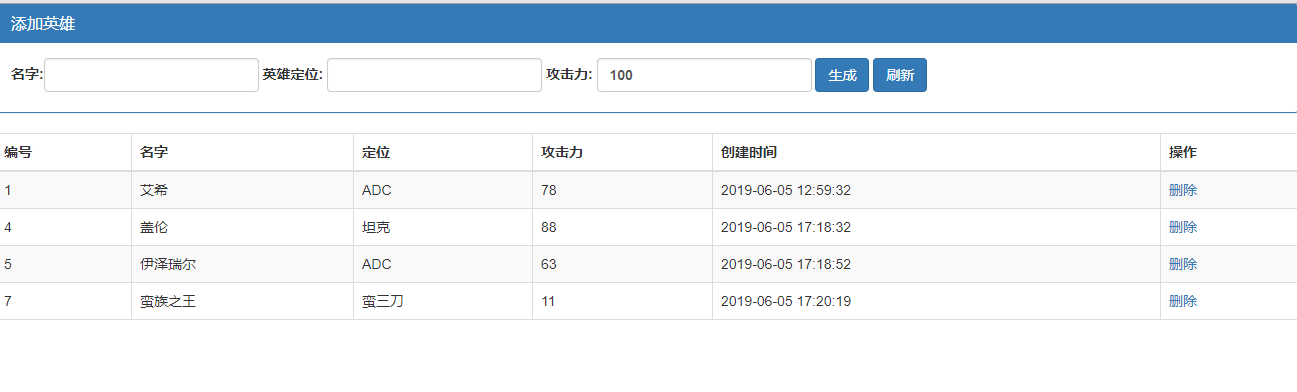
# lib 引入
- vue.js
- vue-resource.js
- bootstrap.min.css
# index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>英雄系统案例</title>
<script src="./lib/vue/vue.js"></script>
<script src="./lib/vue/vue-resource.js"></script>
<link rel="stylesheet" href="./lib/bootstrap/css/bootstrap.min.css">
</head>
<body>
<div id="app">
<div class="panel panel-primary">
<div class="panel-heading">
<h3 class="panel-title">添加英雄</h3>
</div>
<div class="panel-body form-inline">
<label for="name">
名字:<input type="text" name="name" v-model="hero.name" class="form-control">
</label>
<label for="typeName">
英雄定位:
<input type="text" name="typeName" v-model="hero.typeName" class="form-control">
</label>
<label for="atk">
攻击力:
<input name="atk" v-model.number="hero.atk" class="form-control">
</label>
<input class="btn btn-primary" type="button" value="生成" @click="createHero()">
<input class="btn btn-primary" type="button" value="刷新" @click="getAllList()">
</div>
</div>
<table class="table table-bordered table-hover table-striped">
<thead>
<tr>
<th>编号</th>
<th>名字</th>
<th>定位</th>
<th>攻击力</th>
<th>创建时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="item in list" :key='item.id'>
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.typeName }}</td>
<td>{{ item.atk }}</td>
<td>{{ item.createTime | dataFormat('yyyy-MM-dd HH:mm:ss') }}</td>
<td><a href="" @click.prevent="delHero(item.id)">删除</a></td>
</tr>
</tbody>
</table>
</div>
</body>
<script src="./main.js"></script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
# main.js
// vue全局过滤器
Vue.filter('dataFormat', (dataStr, fmt) => {
return dateFormat(new Date(dataStr), fmt)
})
// 时间过滤器
function dateFormat(date, fmt) { //author: meizz
var o = {
"M+": date.getMonth() + 1, //月份
"d+": date.getDate(), //日
"H+": date.getHours(), //小时
"m+": date.getMinutes(), //分
"s+": date.getSeconds(), //秒
"q+": Math.floor((date.getMonth() + 3) / 3), //季度
"S": date.getMilliseconds() //毫秒
};
if (/(y+)/.test(fmt))
fmt = fmt.replace(RegExp.$1, (date.getFullYear() + "").substr(4 - RegExp.$1.length));
for (var k in o)
if (new RegExp("(" + k + ")").test(fmt))
fmt = fmt.replace(RegExp.$1, (RegExp.$1.length == 1) ? (o[k]) : (("00" + o[k]).substr(("" + o[k]).length)));
return fmt;
}
// 设置vue-resource根目录
// 注意,官方已经明确提示了,如果使用该方式配置,在请求时不能使用绝对路径。比如以'/'开头。
Vue.http.options.root = 'http://localhost'
Vue.http.options.emulateJSON = json
var vm = new Vue({
el: '#app',
data: {
hero: {
name: '',
typeName: '',
atk: 100.00
},
list: []
},
created() {
this.getAllList()
},
methods: {
async ajax(url, body, method = 'GET', json = false) {
return new Promise(resolve => {
this.$http({
method: method,
url: url,
headers: {
'Accept': 'application/json;charset=utf-8',
'Content-Type': 'application/json;charset=utf-8',
'Cache-Control': 'no-cache',
'cache': 'no-cache',
},
body: { value: body }
}).then(result => {
const resJson = result.body.value
console.info(resJson)
resolve(resJson)
}).catch(err => {
resolve({ code: 500, data: '', msg: err.toString() })
})
})
},
// 获取列表
async getAllList() {
let result = await this.ajax('hero/get_all_list', {}, 'GET')
this.list = result.data
},
// 创建英雄
async createHero() {
if (this.hero.name === "" || this.hero.typeName === "") {
console.info("请填写参数!")
return
}
let result = await this.ajax('hero/create_hero', this.hero, 'POST')
if (result.code === 1) {
console.info('添加英雄成功!')
this.getAllList()
}
},
// 删除英雄
async delHero(id) {
let result = await this.ajax('hero/del_hero', { id }, 'POST')
if (result.code === 1) {
console.info('删除英雄成功!')
this.getAllList()
}
}
}
})
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91